handlebars
Configure the Handlebars file extension and add custom helpers to your templates.
Usage
Key | Type | Required | Default |
---|---|---|---|
"handlebars" | array | No | [] |
↳ "additional-helpers" | array | No | [] |
↳ "template-extension" | string | No | "tpl" |
↳ "template-directory" | string | No | "tpl" |
The value of the "handlebars"
field is an associative array containing two optional items to configure.
Additional Helpers
Handlebars helpers are methods inside your template files to perform php code and return something for a given input. See the handlebars-php docs for some included helpers, otherwise here’s an example of how to write your own.
Writing
Organizing your helpers is important, as it’s not recommended you write functions directly inside the config.php
file. Creating a helpers/
folder is the way we’d suggest doing this, and then creating individual files for helpers used in different templates.
In my helpers/ShowHelpers.php
file, for example, I wrote this function to generate a rating bar html string from a some given parameters (here’s what it will look like):
# All of your helpers across multiple files should use the same namespace.namespace ThemeHelpers; class ShowHelpers{ public static function ratingBar() { return function ($template, $context, $args, $source) { $params = explode(" ", $args); $r = floatval($context->get($params[0])); $b = $params[1]; $c = $params[2]; $str = ""; foreach (range(1, floor($r * $b)) as $number) { $str .= "$c"; } foreach (range(ceil($r * $b), ($b * 5)) as $number) { $str .= "$c"; } return $str; }; }}
You’ll notice the ratingBar()
function returns a function instead of a value, this is to prevent caching.
You’ll also notice that my $r
variable is defined using $context->get(...)
while $b
and $c
aren’t. This is because the first parameter is the name of a variable, while the second and third are just a number and a string. Using $context->get(...)
will return the value of the variable.
Importing
Since we’re using a custom namespace to declare our functions, we’ll use Composer to import these files automagically. In our composer.json
file:
{ "require": { "open-function-computers-llc/rad-theme-engine": "^1.0" }, "autoload": { "psr-4": { "ThemeHelpers\\": "helpers/" } }}
The key is the name of the namespace followed by two backslashes, and the value is the folder it’s located in.
To install this new namespace into Composer’s autoload.php
file, execute the following command in the base of your theme.
composer dump-autoload -o
Configuring
Now in our config.php
file we can add the following:
return [ "handlebars" => [ "additional-helpers" => [ "ratingBar" => ThemeHelpers\ShowHelpers::ratingBar(), ], ], ...]
Implementing
From now onwards, we can use this new helper in any of our templates by calling it with a #ratingBar
. Here’s an example of a list of TV Shows, each with a rating displayed:
<div class="four-col-grid"> {{#each shows}} <div> <a href="{{url}}"> <img class="full-image" src="{{img}}"> <span>{{title}}</span> </a> <span class="show-item-sub-title"> {{rating}}/5 <!-- Implement my helper --> {{#ratingBar rating 2 ▰}} </span> </div> {{/each}}</div>
Like I mentioned before, rating
is a variable, more specifically a member of show
, that was passed into this template using $site->view(...)
.
Results
Finally, here’s what gets rendered. Yours might look a little nicer, but at least you can see how all the variables came into play. The 2
parameter is what made the bar 10 characters long instead of the normal 5, if that wasn’t apparent.
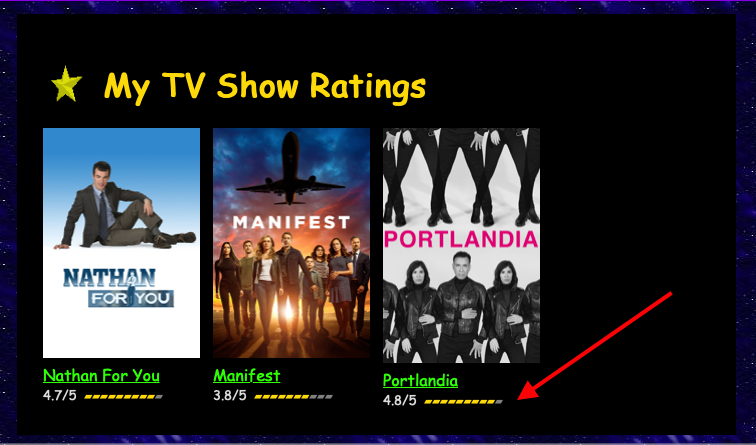
Template Extension
To change your template extension from the default (.tpl
) here are some instructions.
Template Directory
Changing template-directory
will change where handlebars looks for your template files.
Example
This is a theme configuration with a couple additional helpers, a custom .view
template extension, where template files will live in the views
folder instead of tpl
.
return ["handlebars" => [ "additional-helpers" => [ "ratingBar" => ThemeHelpers\ShowHelpers::ratingBar(), "breadcrumbs" => ThemeHelpers\GlobalHelpers::breadcrumbs(), "num" => ThemeHelpers\GlobalHelpers::num(), "asset" => ThemeHelpers\GlobalHelpers::asset(), ], "template-extension" => "view", "template-directory" => "views", ],]